Enhancing Your Next.js App with Tailwind CSS, Redux, and React Optimization Techniques
Learn how to build and optimize a Next.js app with Tailwind CSS for styling, Redux for state management, and tips for enhancing front-end performance.
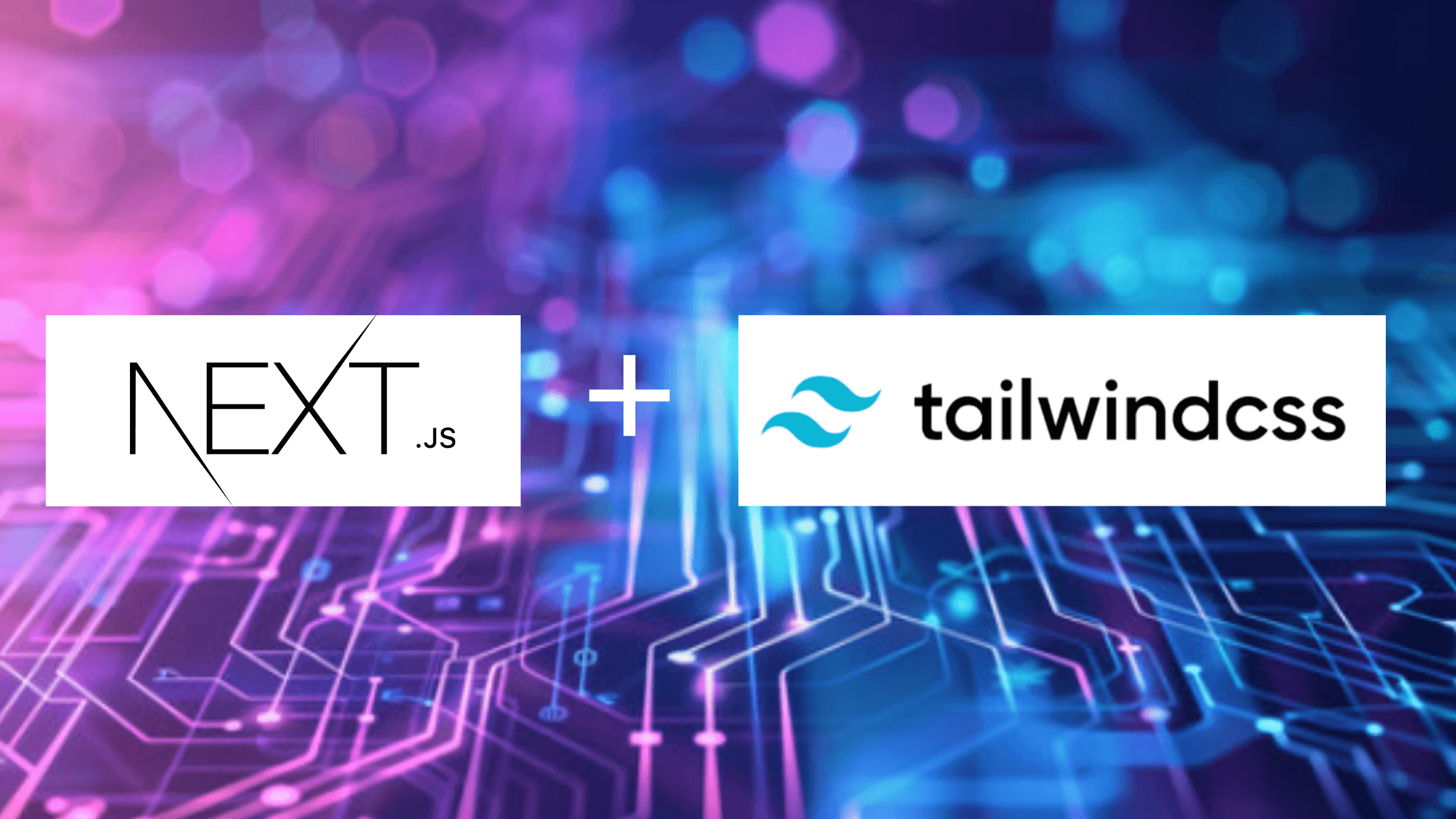
Next.js is a powerful framework that combines React with server-side rendering (SSR) and static site generation, making it a popular choice for building scalable, performant web applications. In this tutorial, we’ll dive into setting up a fullstack Next.js application, but we’ll focus more on the front-end aspects, styling with Tailwind CSS, state management with Redux, and optimizing performance in React apps.
We’ll also briefly cover deploying the app using PM2 for process management and Nginx as a reverse proxy to ensure that your application runs smoothly in production.
Prerequisites
Before we dive in, make sure you have the following:
- A basic Next.js application (if you don’t have one, you can quickly create it using
npx create-next-app
) - Node.js installed locally
- Basic knowledge of React and JavaScript
For the styling part, we’ll use Tailwind CSS, and for state management, Redux. Let’s get started!
1. Setting Up Styling with Tailwind CSS
Tailwind CSS is an excellent utility-first framework that simplifies styling in your React applications. You can quickly build responsive UIs with predefined classes, and its integration with Next.js is seamless.
Step 1: Install Tailwind CSS
In your Next.js project folder, run the following commands to install Tailwind CSS and its dependencies:
npm install -D tailwindcss postcss autoprefixer
npx tailwindcss init -p
This will create a tailwind.config.js
file, where you can configure Tailwind. For now, we’ll stick with the default settings.
Step 2: Configure Tailwind
Update the tailwind.config.js
file to include your content paths:
module.exports = {
content: [
'./pages/**/*.{js,ts,jsx,tsx}',
'./components/**/*.{js,ts,jsx,tsx}',
],
theme: {
extend: {},
},
plugins: [],
}
Next, add Tailwind to your global CSS file (usually styles/globals.css
):
@tailwind base;
@tailwind components;
@tailwind utilities;
Now, you can start using Tailwind’s utility classes directly in your components:
<div className="bg-blue-500 text-white p-4 rounded-lg">
Welcome to my Next.js app styled with Tailwind!
</div>
With this setup, you can easily create responsive layouts and quickly prototype your UIs.
2. Managing State with Redux
State management is crucial in larger applications, and while Next.js provides its own API routes and hooks for managing state, Redux remains a popular choice for handling global state in complex React applications.
Step 1: Install Redux and React-Redux
To add Redux to your Next.js app, install the necessary packages:
npm install redux react-redux @reduxjs/toolkit
We’re using the Redux Toolkit to simplify the process of setting up Redux. Create a store.js
file in the root of your project:
import { configureStore } from '@reduxjs/toolkit'
export const store = configureStore({
reducer: {
// Add your reducers here
},
})
Then, wrap your application with the Provider
in pages/_app.js
to make the store accessible throughout your app:
import { Provider } from 'react-redux'
import { store } from '../store'
function MyApp({ Component, pageProps }) {
return (
<Provider store={store}>
<Component {...pageProps} />
</Provider>
)
}
export default MyApp
Step 2: Create Slices for State
With Redux Toolkit, you can create slices to manage specific pieces of your application state. For example, if you’re managing authentication, create an authSlice.js
:
import { createSlice } from '@reduxjs/toolkit'
export const authSlice = createSlice({
name: 'auth',
initialState: {
user: null,
},
reducers: {
login: (state, action) => {
state.user = action.payload
},
logout: (state) => {
state.user = null
},
},
})
export const { login, logout } = authSlice.actions
export default authSlice.reducer
Add the reducer to your store, and now you can use Redux to handle authentication, user data, or other global states in your app.
3. Performance Optimization in React
Performance is key when building modern web applications. With React and Next.js, there are several ways to ensure your app runs smoothly and loads quickly.
Code Splitting & Lazy Loading
React’s lazy loading allows you to split your code and load components only when needed, reducing initial load times:
import React, { Suspense, lazy } from 'react'
const SomeComponent = lazy(() => import('./SomeComponent'))
function MyApp() {
return (
<Suspense fallback=<div>Loading...</div>>
<SomeComponent />
</Suspense>
)
}
This ensures that large components don’t block the rendering of other elements on your page.
Image Optimization
Next.js includes built-in image optimization, which automatically optimizes images for different screen sizes and formats. Use the next/image
component for best results:
import Image from 'next/image'
<Image
src="/path-to-image.jpg"
alt="Optimized Image"
width={500}
height={300}
/>
Caching and CDN
Leverage caching mechanisms and a CDN to deliver static assets faster. Next.js can automatically generate static pages during build time, which improves performance.
4. Basic Deployment with PM2 and Nginx (Optional)
Once your app is ready for production, you’ll need to deploy it in a stable environment. Here’s a quick look at deploying your app with PM2 and Nginx for process management and reverse proxy, respectively.
Step 1: Install PM2
First, install PM2 globally on your server:
npm install -g pm2
Then, use PM2 to start your Next.js app:
pm2 start npm --name "next-app" -- start
Step 2: Set Up Nginx
Nginx acts as a reverse proxy to route requests from port 80 (HTTP) to your Next.js app running on another port (e.g., 3000). Configure Nginx to forward requests:
server {
listen 80;
server_name your-domain.com;
location / {
proxy_pass http://localhost:3000;
proxy_http_version 1.1;
proxy_set_header Upgrade $http_upgrade;
proxy_set_header Connection 'upgrade';
proxy_set_header Host $host;
proxy_cache_bypass $http_upgrade;
}
}
Restart Nginx, and your app is now live in production.
Conclusion
By combining Next.js with Tailwind CSS for styling and Redux for state management, you can build powerful, performant web applications. For deployment, tools like PM2 and Nginx help ensure your app runs reliably in production.
Stay tuned for more posts on improving React performance, optimizing your build pipeline, and enhancing security in web applications!